JavaScript Interview Questions: Top 50 JavaScript Interview Questions Every Developer Should Know
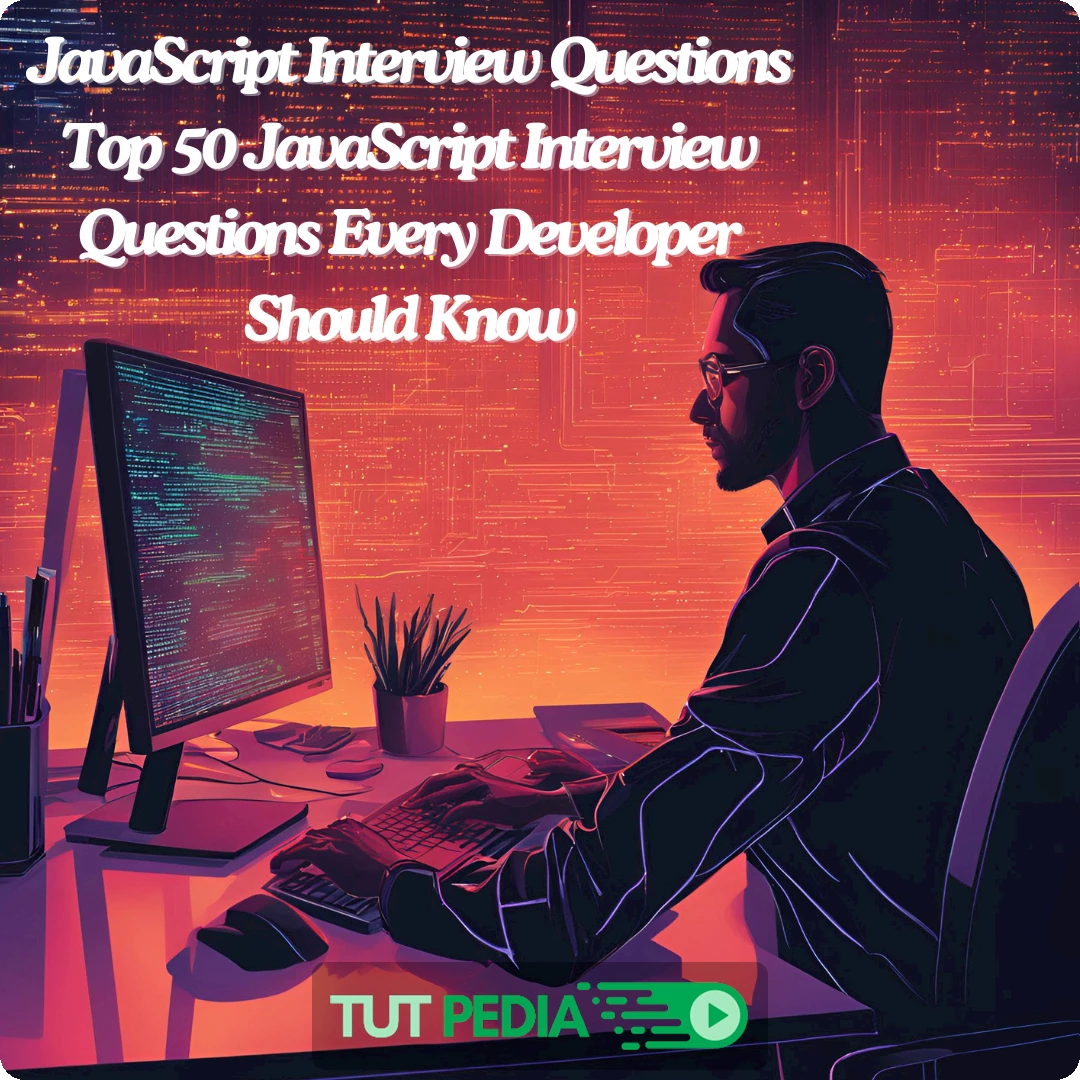
For decades, JavaScript was, first and foremost, a cornerstone of web development. If you know JavaScript, you are halfway through learning to program — it is one of the most popular programming languages and among the most important if you want to make a living as a software developer. If you’re preparing for any technical interview, especially those with a front-end or full-stack track, it makes sense to be up and running with JavaScript.
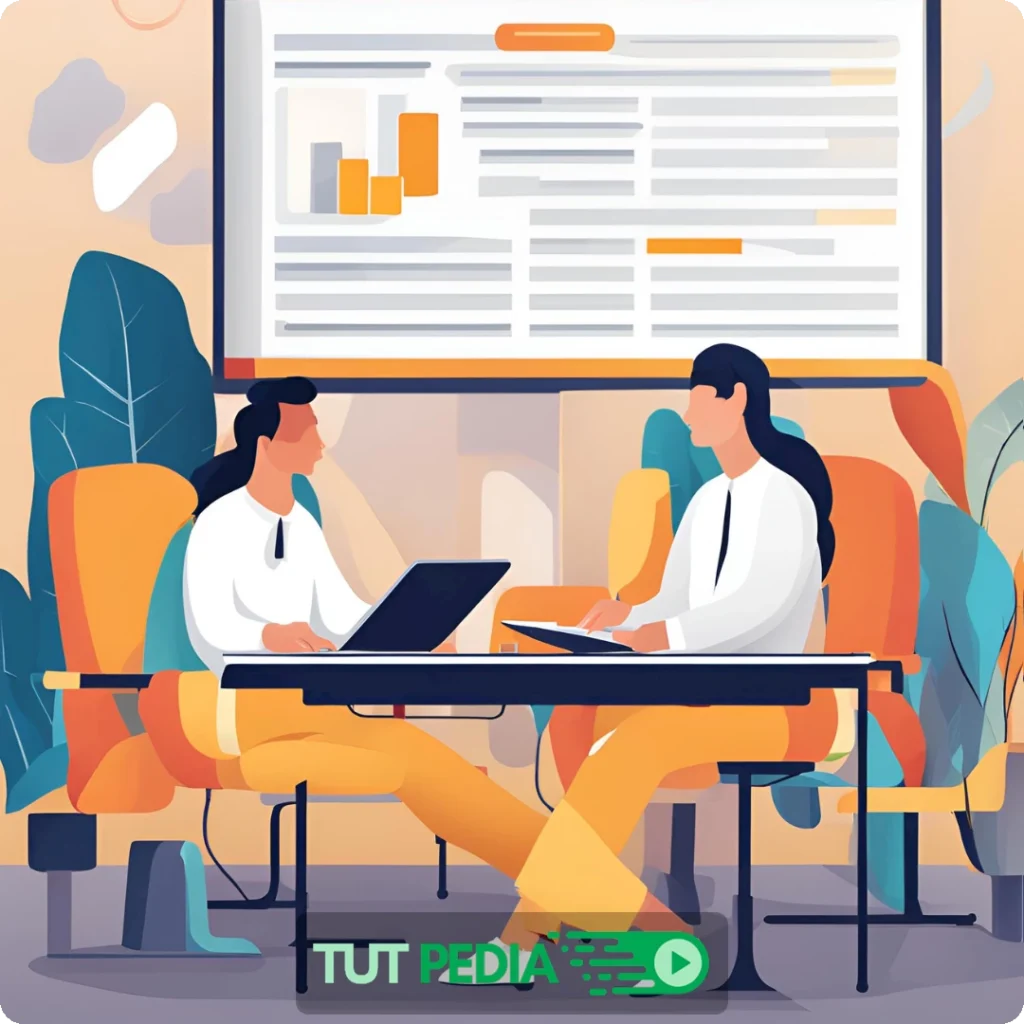
In this blog post, I’ve written about 50 essential questions on JavaScript to ask for answers during the interview. These JavaScript interview questions are for both the beginner or an experienced who wants to bag his or her first job or the seasoned professional looking for a senior role.
If you want to master JavaScript, explore our free JavaScript courses by following this link: Best JavaScript Courses
Why JavaScript Interview Questions Are Important?
JavaScript interview questions will test your knowledge of the language, problem-solving strategy, and programming fundamentals. They are a great way to prepare to talk confidently about closures, promises, and event delegation, so definitively take the time to prepare for such questions.
Key Areas Covered in JavaScript Interview Questions:
- Basic JavaScript Concepts
- Object-Oriented JavaScript
- Asynchronous Programming
- Error Handling
- DOM Manipulation
- JavaScript Design Patterns
- ES6+ Features
I’ll be breaking down the top 50 JavaScript Interview Questions into beginner, intermediate, and advanced levels of questions.
Beginner JavaScript Interview Questions
- What is JavaScript? JavaScript is a dynamic programming language that enhances web page user interactions. It enables the creation of responsive elements like menus, animations, and form validations.
- What is the difference between let, var, and const? These are keywords used to declare variables in JavaScript. var is function-scoped, while let and const are block-scoped. const is used for variables that should not be reassigned.
- Explain JavaScript hoisting. Hoisting is JavaScript’s default behavior of moving declarations (not initializations) to the top of their scope. This means you can use functions and variables before declaring them.
- What is a closure in JavaScript? A closure is a function that remembers its lexical environment, meaning it can access variables from its parent scope even after that parent function has finished executing.
- What is the difference between == and ===? == compares values for equality but ignores type, while === checks for both value and type equality. It’s generally recommended to use === to avoid type coercion.
Intermediate JavaScript Interview Questions
- What is event delegation? Event delegation is a technique where a single event listener is attached to a parent element to manage events triggered by child elements. This is useful for dynamically added elements.
- Explain promises in JavaScript. A promise is an object representing the eventual completion or failure of an asynchronous operation. Promises have three states: pending, fulfilled, and rejected.
- What are arrow functions in JavaScript? Arrow functions, introduced in ES6, provide a more concise syntax for writing functions. They also lexically bind this keyword, which differs from traditional function expressions.
- What is this in JavaScript? The value of this depends on the context of execution. In a method, this refers to the object it belongs to. In a global context, it refers to the global object (e.g., window in browsers).
- How does JavaScript handle asynchronous operations? JavaScript handles asynchronous operations using callbacks, promises, and async/await (introduced in ES8). These techniques allow non-blocking operations, improving performance.
Advanced JavaScript Interview Questions
- What is prototypal inheritance in JavaScript? In JavaScript, objects can inherit properties and methods from other objects. This is achieved through the prototype chain, which allows one object to serve as a template for another.
- What is the difference between call(), apply(), and bind()? These methods allow you to set the value of this inside a function. call() and apply() immediately invoke the function, while bind() returns a new function that can be invoked later. apply() accepts arguments as an array, while call() takes them individually.
- Explain the event loop in JavaScript. The event loop is responsible for executing asynchronous code. It continuously checks and processes the message queue for tasks, ensuring that non-blocking operations like API calls don’t interrupt the main thread.
- What are generators in JavaScript? Generators are special functions that can be paused and resumed. They are useful for handling asynchronous operations more efficiently. A generator function is declared using function* and yields values using the yield keyword.
- What is the purpose of async and await in JavaScript? async and await are syntactic sugar for working with promises. An async function always returns a promise and await pauses the execution until the promise is resolved.
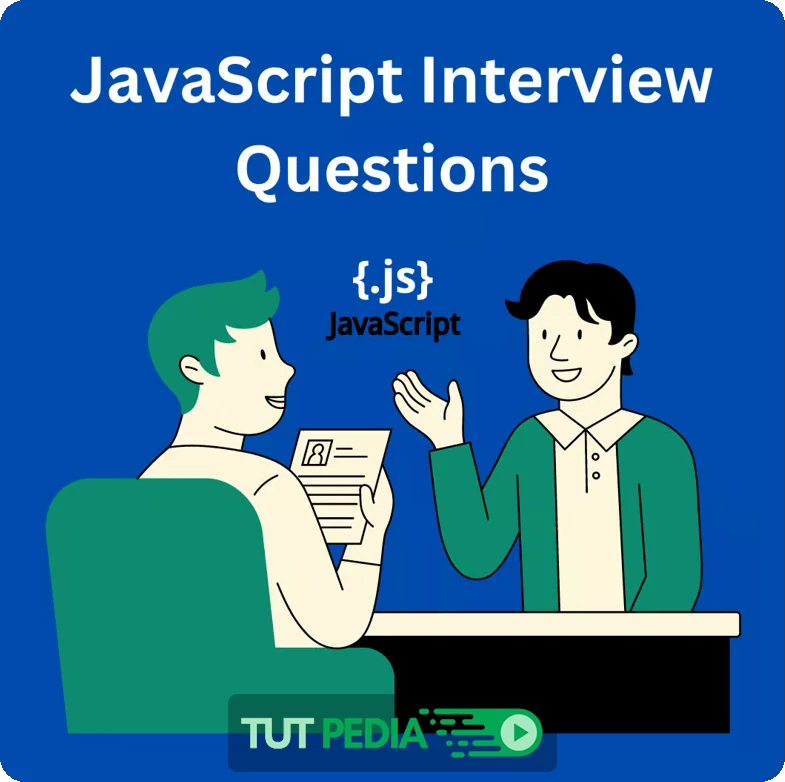
Preparing for JavaScript Interview Questions
Practising these JavaScript Interview Questions and understanding how to apply them in a real-world coding scenario is essential to navigating your next technical interview. Interviewers might also examine your ability to solve coding challenges or do problem-solving exercises, so don’t forget to write clean and efficient code.
Conclusion
These top JavaScript Interview Questions will help you if you’re a beginner or experienced developer and will give you an edge in your next job interview. I remember that preparation matters, and knowing the ‘How’ behind the scenes helps you out of other candidates.
Because we care, we prepared these JavaScript questions to get you started riddling and help you land that dream developer job.